Congratulations on your quest to unravel the secrets of reversing strings in Python! It’s akin to orchestrating a captivating dance where characters pirouette, and the last becomes the first. Python, like a seasoned choreographer, enables you to rearrange the characters in a string effortlessly. Let’s explore this fundamental operation through five distinct techniques, with clear examples for each.
Understanding String Reversal in Python
String reversal involves flipping the order of characters in a string. It’s a common programming task applicable in diverse scenarios, from text processing to cryptography. Python, being versatile, offers several methods for achieving this.
Technique 1: Slicing Magic
Slicing is Python’s wand for extracting specific portions of a sequence, including strings. To reverse a string using slicing, employ the syntax `a_string[::-1]`. This concise one-liner efficiently reverses the entire string.
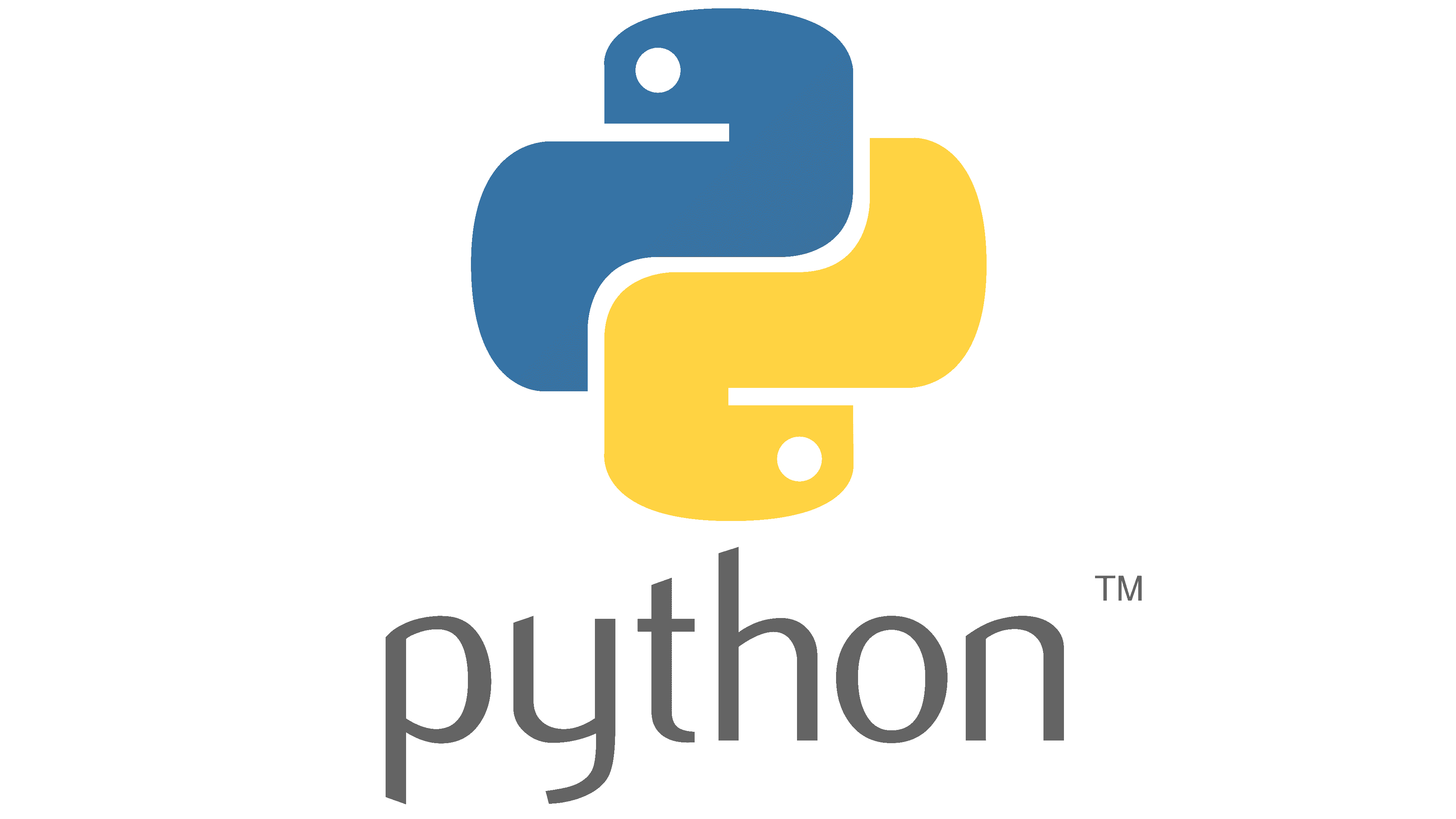
Code:-
original_string = “Hello”
reversed_string = original_string[::-1]
print(reversed_string)
Output: “olleH”
Slicing shines with its simplicity, readability, and performance, making it the recommended choice for string reversal.
Technique 2: Looping Marvels
Using a For Loop
A for loop provides a fundamental approach to reverse a string. It iterates through the original string from the last character to the first, building the reversed string along the way.
Code:-
original_string = “Hello”
reversed_string = “”
for char in original_string:
reversed_string = char + reversed_string
print(reversed_string)
Output: “olleH”
Using a While Loop
Similarly, a while loop achieves the same result. It initializes an index to the last character’s position and traverses the string in reverse order.
Code:-
original_string = “Hello”
reversed_string = “”
index = len(original_string) – 1
while index >= 0:
reversed_string += original_string[index]
index -= 1
print(reversed_string)
Output: “olleH”
Technique 3: Recursive Elegance
Recursion breaks down the string into smaller parts until it reaches the base case of a single character. It then reconstructs the reversed string.
Code:-
def reverse_string(input_str):
if len(input_str) == 1:
return input_str
else:
return input_str[-1] + reverse_string(input_str[:-1])
original_str = “Python”
reversed_str = reverse_string(original_str)
print(reversed_str)
Output: “nohtyP”
Technique 4: Functional Simplicity
Using a function to reverse a string adds organization and reusability to your code. The function takes an input string, iterates through it, and returns the reversed string.
Code:-
def reverse_string(input_string):
reversed_str = “”
for char in input_string:
reversed_str = char + reversed_str
return reversed_str
original_string = “Hello, World!”
reversed_result = reverse_string(original_string)
print(reversed_result)
Output: “!dlroW ,olleH”
Technique 5: List Comprehension Charm
List comprehension offers a concise and elegant way to reverse a string. It creates a list of characters in reverse order and joins them to form the reversed string.
Code:-
original_string = “Python”
reversed_list = [char for char in original_string[::-1]]
reversed_string = ”.join(reversed_list)
print(reversed_string)
Output: “nohtyP”
Choosing the Best Technique
The most efficient technique depends on your specific needs. For simplicity and speed, slicing is often recommended. Loops and recursion are beneficial when additional logic or complex transformations are required during reversal.
In the vast landscape of Python, where flexibility reigns supreme, pick the technique that aligns with your task or coding preferences. Whether you’re orchestrating a dance of characters or handling cryptography, mastering these string reversal techniques empowers you in the Pythonic realm.
In conclusion, string reversal in Python is a versatile skill with applications ranging from fundamental text manipulations to intricate cryptographic tasks. Each technique presented here carries its own charm, and your choice depends on the context of your programming endeavor. Happy coding!